In this article we are going to see how we can use Cypress and Cucumber for effective BDD style test automation.
Step – 1: First thing first, create a folder: cypress-bdd-example
Step – 2: Open Terminal and navigate to the folder. Time to install Cypress in that folder. So, run the below command –
npm install cypress
Step – 3: In order to combine Cypress with Cucumber, we need to pull a package, cypress-cucumber-preprocessor
npm install cypress-cucumber-preprocessor
Step – 4: Once these two packages are installed, lets now lets call the cucumber-preprocessor in the index.js file
const cucumber = require('cypress-cucumber-preprocessor').default
module.exports = (on, config) => {
on('file:preprocessor', cucumber())
}
Step – 5: Lets bind the step definitions by adding the below command in packages.json file
"cypress-cucumber-preprocessor": {
"nonGlobalStepDefinitions": true
}
Step – 6: The features and the step definitions hierarchy is a bit different from other languages. The step definitions file should be located inside a folder and the best practice is to have the folder name, feature file name and the step definitions file name as one and the same.

BDD-Cypress
Step – 7: In this article we will use Swag Labs (https://saucedemo.com) website.
Let’s create a feature file with a scenario in it. Create a feature file named – login.feature
Feature: Login Feature
As a valid customer
In order to purchase items
I want to login successfully to Swag Labs
Scenario: Login Validation
Given I am in the Swag Labs login page
When I enter valid credentials
Then I should be able to login successfully
Step – 8: Now, let’s create a step definitions class under login folder – login.js.
Please note, you need to import {Given, When, Then} from cypress-cucumber-preprocessor/steps package
import {Given, When, Then} from "cypress-cucumber-preprocessor/steps"
So, our Step Definitions class would look like –
import {Given, When, Then} from "cypress-cucumber-preprocessor/steps"
Given('I am in the demo site',()=>{
cy.visit("https://www.saucedemo.com/index.html");
})
When('I enter valid credentials',()=>{
cy.get('[data-test=username]').type("standard_user");
cy.get('[data-test=password]').type("secret_sauce");
cy.get('.btn_action').click();
})
Then('I should be able to login successfully',()=>{
cy.get('.bm-burger-button > button').click();
cy.get('#logout_sidebar_link').click();
})
Step -9: Bingo! We just successfully wrote a simple BDD fashioned test using Cypress.io. Now, its time to launch the Test Runner and execute the tests. As usual, execute the below command to invoke Test Runner
cypress-bdd-example giridhar$ npx cypress open
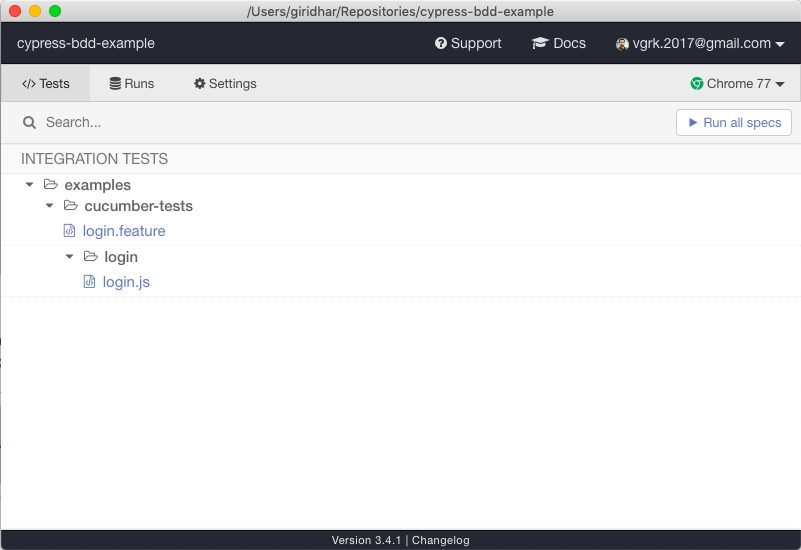
Let’s see it in action –
Code:
You can get the code base for this article from GitHub
Awesome
LikeLike
Thanks Narayanan Palani…
LikeLike
Thanks for making it so simple for us. Goodonya mate!
LikeLike